Neste programa vamos utilizar o JavaScript para calcular Unidades de Energia, tais como: watts, kilowatts, decibéis, BTU etc.
São 10 categorias. Para saber o equivalente de uma unidade de energia em outra, basta informar na caixa de texto correspondente
e clicar no botão Conver-ter.
Então vejamos como fazer isso:
• Primeiro Passo:
Inicialmente abra o seu editor de HTML, como o Notepad++, por exemplo, e em seguida, selecione a linguagem de programação
que você vai utilizar, por exemplo HTML, CSS, JavaScript.
• Segundo Passo:
Copie o código CSS abaixo, e em seguida, cole no seu Editor de HTML entre as tags <head>.... </head>.
Código CSS:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css">
<style>
@media all and (max-width: 576px) {
.calc2 input[type=text], .calc2 input[type=number] { width:100%; padding:5px; }
.calc2 tr td:nth-child(2) { width:160px; width:calc(100% - 140px); }
.calc2 tr td:nth-child(3) { width: 45px; }
}
</style>
• Terceiro Passo:
Copie o código HTML abaixo e, em seguida, cole no seu Editor de HTML entre as tags <body>... </body>.
Código HTML:
<div id="lcol" class="d-flex justify-content-center">
<div id="doc" style="background-color: #f9563a;">
<h3 class="text-center text-light">
Conversor de Unidades de Energia</h3>
<p class="text-center">
Informe o valor correspondente na Caixa de Texto<br> e pressione o Botão <i>Converter</i>:</p>
<form name="calcform" autocomplete="off" class="m-3">
<table class="calc2">
<tr>
<td><label for="mw">Miliwatts:</label></td>
<td><input type="number" min="0" step="any" id="mw" name="mw" autofocus tabindex="1"></td>
<td class="mathsymbol">mW</td>
<td><input onclick="Convert_mW()" type="button" value="Converter" tabindex="2" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="w">Watts:</label></td>
<td><input type="number" min="0" step="any" id="w" name="w" tabindex="3"></td>
<td class="mathsymbol">W</td>
<td><input onclick="Convert_W()" type="button" value="Converter" tabindex="4" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="kw">Kilowatts:</label></td>
<td><input type="number" min="0" step="any" min="0" step="any" id="kw" name="kw" tabindex="5"></td>
<td class="mathsymbol">kW</td>
<td><input onclick="Convert_kW()" type="button" value="Converter" tabindex="6" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="mew">Megawatts:</label></td>
<td><input type="number" min="0" step="any" id="mew" name="mew" tabindex="7"></td>
<td class="mathsymbol">MW</td>
<td><input onclick="Convert_MW()" type="button" value="Converter" tabindex="8" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="gw">Gigawatts:</label></td>
<td><input type="number" min="0" step="any" id="gw" name="gw" tabindex="9"></td>
<td class="mathsymbol">GW</td>
<td><input onclick="Convert_GW()" type="button" value="Converter" tabindex="10" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="dbm">Decibel-Miliwatts:</label></td>
<td><input type="number" min="0" step="any" id="dbm" name="dbm" tabindex="11"></td>
<td class="mathsymbol">dBm</td>
<td><input onclick="Convert_dBm()" type="button" value="Converter" tabindex="12" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="dbw">Decibel-Watts:</label></td>
<td><input type="number" min="0" step="any" id="dbw" name="dbw" tabindex="13"></td>
<td class="mathsymbol">dBW</td>
<td><input onclick="Convert_dBW()" type="button" value="Converter" tabindex="14" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="hpm">HorsePower (mecânica):</label></td>
<td><input type="number" min="0" step="any" id="hpm" name="hpm" tabindex="15"></td>
<td class="mathsymbol">hp(I)</td>
<td><input onclick="Convert_hpM()" type="button" value="Converter" tabindex="16" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="hpe">HorsePower (elétrica):</label></td>
<td><input type="number" min="0" step="any" id="hpe" name="hpe" tabindex="17"></td>
<td class="mathsymbol">hp(E)</td>
<td><input onclick="Convert_hpE()" type="button" value="Converter" tabindex="18" class="btn-sm btn-success"></td>
</tr>
<tr>
<td><label for="btuhr">BTU/hr:</label></td>
<td><input type="number" min="0" step="any" id="btuhr" name="btuhr" tabindex="19"></td>
<td class="mathsymbol">BTU<sub><span style="font-size:10px">IT</span></sub>/hr</td>
<td><input onclick="Convert_BTUhr()" type="button" value="Converter" tabindex="20" class="btn-sm btn-success"></td>
</tr>
<tr>
<td> </td>
<td><br><center><input type="reset" value="Limpa tudo" class="btn btn-warning"></center></td>
<td> </td>
<td> </td>
</tr>
</table>
</form>
</div>
</div>
• Quarto Passo:
Copie o código JavaScript abaixo e cole no seu editor de HTML logo acima da tag </body>.
Código JavaScript:
<script>
function setfocus() {
document.calcform.mw.focus();
}
function Round(x,res)
{
//check 33 GW
y = parseFloat(x);
res = Math.pow(10,res);
y = Math.round(y*res)/res;
//y = roundnum(x,p)
return y;
}
function Convert_mW()
{
mw = document.calcform.mw.value;
if( mw=="" )
return;
mw = parseFloat(mw);
document.calcform.w.value = Round(mw/1e3, 12);
document.calcform.kw.value = Round(mw/1e6, 12);
document.calcform.mew.value = Round(mw/1e9, 12);
document.calcform.gw.value = Round(mw/1e12,12);
document.calcform.dbm.value = Round(10*Math.log(mw)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(mw)*Math.LOG10E-30, 12);
document.calcform.hpm.value = Round(mw*1e-3/745.699872, 12);
document.calcform.hpe.value = Round(mw*1e-3/746, 12);
document.calcform.btuhr.value = Round(mw*1e-3/0.29307107, 12);
}
function Convert_W()
{
w = document.calcform.w.value;
if( w=="" )
return;
w = parseFloat(w);
document.calcform.mw.value = Round(w*1e3, 12);
document.calcform.kw.value = Round(w/1e3, 12);
document.calcform.mew.value = Round(w/1e6, 12);
document.calcform.gw.value = Round(w/1e9, 12);
document.calcform.dbm.value = Round(10*Math.log(w*1000)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(w)*Math.LOG10E, 12);
document.calcform.hpm.value = Round(w/745.699872, 12);
document.calcform.hpe.value = Round(w/746, 12);
document.calcform.btuhr.value = Round(w/0.29307107, 12);
}
function Convert_kW()
{
kw = document.calcform.kw.value;
if( kw=="" )
return;
kw = parseFloat(kw);
document.calcform.mw.value = Round(kw*1e6, 12);
document.calcform.w.value = Round(kw*1e3, 12);
document.calcform.mew.value = Round(kw/1e3, 12);
document.calcform.gw.value = Round(kw/1e6, 12);
document.calcform.dbm.value = Round(10*Math.log(kw*1e6)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(kw*1e3)*Math.LOG10E, 12);
document.calcform.hpm.value = Round(kw*1e3/745.699872, 12);
document.calcform.hpe.value = Round(kw*1e3/746, 12);
document.calcform.btuhr.value = Round(kw*1e3/0.29307107, 12);
}
function Convert_MW()
{
mw = document.calcform.mew.value;
if( mw=="" )
return;
mw = parseFloat(mw);
document.calcform.mw.value = Round(mw*1e9, 12);
document.calcform.w.value = Round(mw*1e6, 12);
document.calcform.kw.value = Round(mw*1e3, 12);
document.calcform.gw.value = Round(mw/1e3, 12);
document.calcform.dbm.value = Round(10*Math.log(mw*1e9)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(mw*1e6)*Math.LOG10E, 12);
document.calcform.hpm.value = Round(mw*1e6/745.699872, 12);
document.calcform.hpe.value = Round(mw*1e6/746, 12);
document.calcform.btuhr.value = Round(mw*1e6/0.29307107, 12);
}
function Convert_GW()
{
gw = document.calcform.gw.value;
if( gw=="" )
return;
gw = parseFloat(gw);
document.calcform.mw.value = Round(gw*1e12, 12);
document.calcform.w.value = Round(gw*1e9, 12);
document.calcform.kw.value = Round(gw*1e6, 12);
document.calcform.mew.value = Round(gw*1e3, 12);
document.calcform.dbm.value = Round(10*Math.log(gw*1e12)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(gw*1e9)*Math.LOG10E, 12);
document.calcform.hpm.value = Round(gw*1e9/745.699872, 12);
document.calcform.hpe.value = Round(gw*1e9/746, 12);
document.calcform.btuhr.value = Round(gw*1e9/0.29307107, 12);
}
function Convert_dBm()
{
dbm = document.calcform.dbm.value;
if( dbm=="" )
return;
dbm = parseFloat(dbm);
mw = Math.pow(10,(dbm/10));
document.calcform.mw.value = Round(mw, 12);
document.calcform.w.value = Round(mw/1e3, 12);
document.calcform.kw.value = Round(mw/1e6, 12);
document.calcform.mew.value = Round(mw/1e9, 12);
document.calcform.gw.value = Round(mw/1e12,12);
document.calcform.dbw.value = Round(dbm-30, 12);
document.calcform.hpm.value = Round(mw*1e-3/745.699872, 12);
document.calcform.hpe.value = Round(mw*1e-3/746, 12);
document.calcform.btuhr.value = Round(mw*1e-3/0.29307107, 12);
}
function Convert_dBW()
{
dbw = document.calcform.dbw.value;
if( dbw=="" )
return;
dbw = parseFloat(dbw);
w = Math.pow(10,(dbw/10));
document.calcform.mw.value = Round(w*1e3, 12);
document.calcform.w.value = Round(w, 12);
document.calcform.kw.value = Round(w/1e3, 12);
document.calcform.mew.value = Round(w/1e6, 12);
document.calcform.gw.value = Round(w/1e9, 12);
document.calcform.dbm.value = Round(dbw+30,12);
document.calcform.hpm.value = Round(w/745.699872, 12);
document.calcform.hpe.value = Round(w/746, 12);
document.calcform.btuhr.value = Round(w/0.29307107, 12);
}
function Convert_hpM()
{
hpm = document.calcform.hpm.value;
if( hpm=="" ) return;
w = hpm*745.699872;
w = parseFloat(w);
document.calcform.mw.value = Round(w*1e3, 12);
document.calcform.w.value = Round(w , 12);
document.calcform.kw.value = Round(w/1e3, 12);
document.calcform.mew.value = Round(w/1e6, 12);
document.calcform.gw.value = Round(w/1e9, 12);
document.calcform.dbm.value = Round(10*Math.log(w*1000)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(w)*Math.LOG10E, 12);
document.calcform.hpe.value = Round(w/746, 12);
document.calcform.btuhr.value = Round(w/0.29307107, 12);
}
function Convert_hpE()
{
hpe = document.calcform.hpe.value;
if( hpe=="" ) return;
w = hpe*746;
w = parseFloat(w);
document.calcform.mw.value = Round(w*1e3, 12);
document.calcform.w.value = Round(w , 12);
document.calcform.kw.value = Round(w/1e3, 12);
document.calcform.mew.value = Round(w/1e6, 12);
document.calcform.gw.value = Round(w/1e9, 12);
document.calcform.dbm.value = Round(10*Math.log(w*1000)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(w)*Math.LOG10E, 12);
document.calcform.hpm.value = Round(w/745.699872, 12);
document.calcform.btuhr.value = Round(w/0.29307107, 12);
}
function Convert_BTUhr()
{
btuhr = document.calcform.btuhr.value;
if( btuhr=="" ) return;
w = btuhr*0.29307107;
w = parseFloat(w);
document.calcform.mw.value = Round(w*1e3, 12);
document.calcform.w.value = Round(w , 12);
document.calcform.kw.value = Round(w/1e3, 12);
document.calcform.mew.value = Round(w/1e6, 12);
document.calcform.gw.value = Round(w/1e9, 12);
document.calcform.dbm.value = Round(10*Math.log(w*1000)*Math.LOG10E, 12);
document.calcform.dbw.value = Round(10*Math.log(w)*Math.LOG10E, 12);
document.calcform.hpm.value = Round(w/745.699872, 12);
document.calcform.hpe.value = Round(w/746, 12);
}
//-->
function setfocus() {
document.calcform.x.focus();
}
function calc() {
x = document.calcform.x.value;
y = convert(x);
y = roundresult(y);
document.calcform.y.value = y;
}
function calctest() {
x = document.calcform.x.value;
y = convert(x);
//y = roundresult(y);
y = roundresult1(y);
document.calcform.y.value = y;
}
function calc3() {
x1 = document.calcform.x1.value;
x2 = document.calcform.x2.value;
y = convert(x1,x2);
y = roundresult(y);
document.calcform.y.value = y;
}
function calc4() {
x1 = document.calcform.x1.value;
x2 = document.calcform.x2.value;
x3 = document.calcform.x3.value;
y = convert(x1,x2,x3);
y = roundresult(y);
document.calcform.y.value = y;
}
function calc5() {
x = document.calcform.x.value;
y = convert1(x);
y = roundresult(y);
document.calcform.y1.value = y;
y = convert2(x);
y = roundresult(y);
document.calcform.y2.value = y;
}
/*
function calcbase(b1,b2) {
x = document.calcform.x.value;
document.calcform.y.value = convertbase(x,b1,b2);
}
function calcbase2() {
x = document.calcform.x.value;
y = convert(x);
document.calcform.y.value = y;
}
*/
function roundresult(x) {
y = parseFloat(x);
y = roundnum(y,10);
return y;
}
function roundnum(x,p) {
var i;
var n=parseFloat(x);
var m=n.toPrecision(p+1);
var y=String(m);
i=y.indexOf('e');
if( i==-1 ) i=y.length;
j=y.indexOf('.');
if( i>j && j!=-1 )
{
while(i>0)
{
if(y.charAt(--i)=='0')
y = removeAt(y,i);
else
break;
}
if(y.charAt(i)=='.')
y = removeAt(y,i);
}
return y;
}
function removeAt(s,i) {
s = s.substring(0,i)+s.substring(i+1,s.length);
return s;
}
</script>
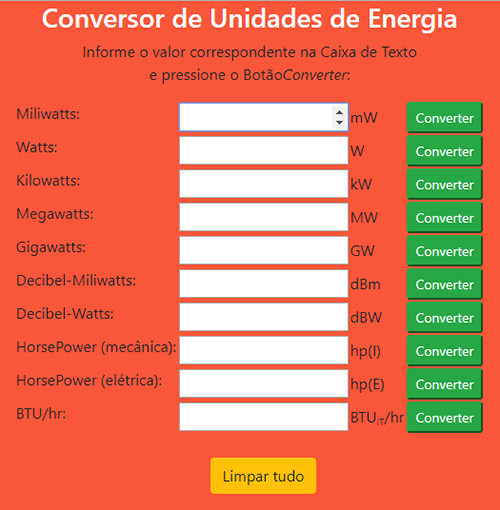
Salve esse arquivo como: CalculaUnidadesEnergia.html.
Execute no navegador clicando no botão abaixo e veja se está igual ao da imagem acima:
|